Intro
I tried to manage my ASP.NET Core & Angular projects with Azure Repos.And I wrote some tests of HttpClient for Angular.
I added pipelines to execute on pulling request.
Prepare
I created new projects.Because I had wanted to the simple projects, so I used empty template for creating ASP.NET Core project.
dotnet new empty -n AspNetCoreAngularSampleAnd I added an Angular project.
cd AspNetCoreAngularSample ng new client-appsI changed csproj, Startup.cs, angular.json and etc. for using JavaScript service as same as last time.
Create Azure DevOps project
I added project to Azure DevOps as same name.When I had opened Repos, because there had been no projects, so I only could links for cloning or pushing the project.
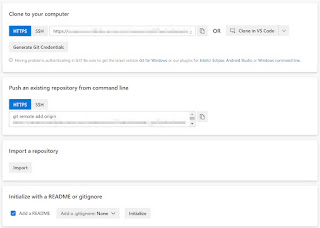
I added remote repository to the ASP.NET Core & Angular projects.
git remote add origin https://example@dev.azure.com/example/AspNetCoreAngularSample/_git/AspNetCoreAngularSample git push -u origin --all
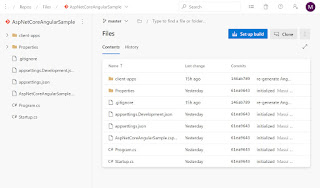
After all, I added a local branch and checked out.
git checkout -b dev/first-page origin/master git push origin dev/first-page
Add Angular test
First, I added test codes for Angular HttpClient.first-page.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { Observable, throwError } from 'rxjs';
import {catchError, map} from 'rxjs/operators';
import { Product } from '../products/product';
@Injectable({
providedIn: 'root'
})
export class FirstPageService {
constructor(private httpClient: HttpClient) { }
public getProducts(): Observable<Array<Product>> {
return this.httpClient.get<Array<Product>>(
`http://localhost:5000/products`)
.pipe(
catchError(FirstPageService.handleError)
)
}
private static handleError(error: HttpErrorResponse) {
console.error(error);
// Do something
return throwError(error.message);
}
}
first-page.service.spec.ts
import { TestBed } from '@angular/core/testing';
import { FirstPageService } from './first-page.service';
import { HttpClient } from '@angular/common/http';
import { HttpClientTestingModule, HttpTestingController } from '@angular/common/http/testing';
describe('FirstPageService', () => {
let httpClient: HttpClient;
let testingController: HttpTestingController;
let service: FirstPageService;
// prepared for the test
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule]
});
// got instances with TestBed
// It also resolved dependencies
httpClient = TestBed.get(HttpClient);
testingController = TestBed.get(HttpTestingController);
service = TestBed.get(FirstPageService);
});
// test spec
it('should get products', () => {
service.getProducts()
.subscribe(products => {
expect(products[0]).toEqual({ id: 1, name: 'Hello'});
});
// When the test target had accessed http://localhost:5000/products,
// HttpTestingController could return mock data.
const request = testingController.expectOne('http://localhost:5000/products');
expect(request.request.method).toBe('GET');
request.flush([{ id: 1, name: 'Hello'}]);
});
// check the result
afterEach(() => {
testingController.verify();
})
});
This time, I had written very simple test.Next time, I would write more.
Try test on the local PC
ng test
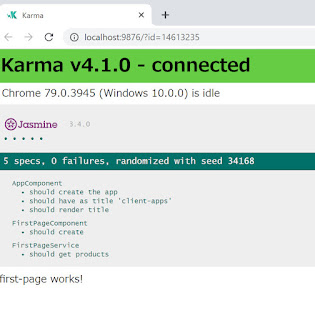
Good :)
Resources
Angular - TestingAngular - HttpClient
ng-book
Try test with Azure Pipelines (Failed)
I had pushed the test to remote repository and added pipeline to run test on the project was built.I had thought the situation had been same as local.
So I added the yaml file like below.
azure-pipelines.yml
trigger:
- master
pool:
vmImage: 'ubuntu-latest'
steps:
- task: NodeTool@0
inputs:
versionSpec: '10.x'
displayName: 'Install Node.js'
- script: |
npm install
npm run test
workingDirectory: client-apps
displayName: 'npm install and test'
YAML schema - Azure Pipelines | Microsoft DocsResult
The test was failed.I got two problems.
1. The error was occurred by Karma
2. The test couldn't be finished
1. The error was occurred by Karma
Because by default, Karma(Test runner) would connect the Chrome to display the test result.But when the test had executed by Azure pipeline, it couldn't do that.
So I had to change the Karma settings to use the ChromeHeadless instead of the Chrome.
I could edit the karma.config.js.
karma.config.js
module.exports = function (config) {
config.set({
...
reporters: ['progress', 'kjhtml'],
port: 9876,
colors: true,
logLevel: config.LOG_INFO,
autoWatch: true,
browsers: ['ChromeHeadless'],
singleRun: false,
restartOnFileChange: true
});
};
I also could add the option to the test command.ng test --browsers=ChromeHeadless
2. The test couldn't be finished
By default, the Karma kept watching until it would be terminated.So when I had run test, I had to make it stopped.
karma.config.js
module.exports = function (config) {
config.set({
...
reporters: ['progress', 'kjhtml'],
port: 9876,
colors: true,
logLevel: config.LOG_INFO,
autoWatch: true,
browsers: ['ChromeHeadless'],
singleRun: true,
restartOnFileChange: true
});
};
I also could add the option to the test command.ng test --watch=falseBecause I wanted to test with default settings on local environment, I added the test command with the options in package.json.
package.json
{
"name": "client-apps",
"version": "0.0.0",
"scripts": {
"ng": "ng",
"start": "ng serve",
"build": "ng build",
"test": "ng test",
"citest": "ng test --code-coverage --watch=false --browsers=ChromeHeadless --reporters=progress",
"lint": "ng lint",
"e2e": "ng e2e"
},
...
azure-pipelines.yml
trigger:
- master
pool:
vmImage: 'ubuntu-latest'
steps:
- task: NodeTool@0
inputs:
versionSpec: '10.x'
displayName: 'Install Node.js'
- script: |
npm install
npm run citest
workingDirectory: client-apps
displayName: 'npm install and test'
Angular unit testing in Azure DevOps + SonarQube - Lucas Moura Veloso - MediumFinally, I succeeded running test.
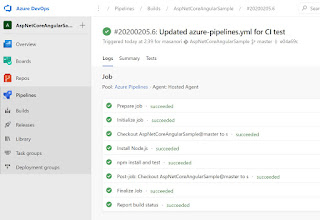
コメント
コメントを投稿